Description:
1. In Design View Coding, add the Javascript Code in Head section to make an integration with Google Maps API Script.
<script src="https://maps.googleapis.com/maps/api/js?sensor=false&libraries=places"
type="text/javascript"></script>
2. Add the Source and Destination functions inside script tags, which is indicating the locations
function Source() {
try {
var input = document.getElementById('TextBox1');
var autocomplete = new google.maps.places.Autocomplete(input);
autocomplete.setTypes('changetype-geocode');
}
catch (err) {
// exception catching here
}
}
function Destination() {
try {
var input = document.getElementById('TextBox2');
var autocomplete = new google.maps.places.Autocomplete(input);
autocomplete.setTypes('changetype-geocode');
}
catch (err) {
// exception catching here;
}
}
3. Add dom event Listner Load function in window
google.maps.event.addDomListener(window, 'load', Source);
google.maps.event.addDomListener(window, 'load', Destination);
4. Finally add these codes, in thecode behind page of Default.aspx.cs which uses Google Maps distancematrix webservice api to get the distance between the two or more Address, city Locations as well as Travelling Time of the two end points in different mode, Here I have used Vehicle Type mode as Bus;
//Travel mode as Bus
public void google_Distance_TravelTime_BusMode()
{
//Declare variable to store XML result
string xmlResult = null;
//Pass request to google api with orgin and destination details
HttpWebRequest request = (HttpWebRequest)WebRequest.Create("http://maps.googleapis.com/maps/api/distancematrix/xml?origins=" + TextBox1.Text + "&destinations=" + TextBox2.Text + "&mode=Bus&language=us-en&sensor=false");
HttpWebResponse response = (HttpWebResponse)request.GetResponse();
//Get response as stream from httpwebresponse
StreamReader resStream = new StreamReader(response.GetResponseStream());
//Create instance for xml document
XmlDocument doc = new XmlDocument();
//Load response stream in to xml result
xmlResult = resStream.ReadToEnd();
//Load xmlResult variable value into xml documnet
doc.LoadXml(xmlResult);
string output = "";
try
{
//Get specified element value using select single node method and verify it return OK (success ) or failed
if (doc.DocumentElement.SelectSingleNode("/DistanceMatrixResponse/row/element/status").InnerText.ToString().ToUpper() != "OK")
{
Label2.ForeColor = System.Drawing.Color.Red;
Label2.Text = "Invalid City Name please try again";
return;
}
//Get DistanceMatrixResponse element and its values
XmlNodeList xnList = doc.SelectNodes("/DistanceMatrixResponse");
foreach (XmlNode xn in xnList)
{
if (xn["status"].InnerText.ToString() == "OK")
{
//Form a table and bind it orgin, destination place and return distance value, approximate duration
output = "<table align='center' width='600' cellpadding='0' cellspacing='0'>";
output += "<tr><td height='60' colspan='2' align='center'><b>Travel Details</b></td>";
output += "<tr><td height='40' width='30%' align='left'>Source</td><td align='left'>" + xn["origin_address"].InnerText.ToString() + "</td></tr>";
output += "<tr><td height='40' align='left'>Destination</td><td align='left'>" + xn["destination_address"].InnerText.ToString() + "</td></tr>";
output += "<tr><td height='40' align='left'>Travel Duration(apprx.) </td><td align='left'>" + doc.DocumentElement.SelectSingleNode("/DistanceMatrixResponse/row/element/duration/text").InnerText + "</td></tr>";
output += "<tr><td height='40' align='left'>Distance</td><td align='left'>" + doc.DocumentElement.SelectSingleNode("/DistanceMatrixResponse/row/element/distance/text").InnerText + "</td></tr>";
output += "</table>";
//finally bind it in the result label control
Label1.ForeColor = System.Drawing.Color.Green;
Label1.Text = doc.DocumentElement.SelectSingleNode("/DistanceMatrixResponse/row/element/distance/text").InnerText;
Label2.ForeColor = System.Drawing.Color.Green;
Label2.Text = doc.DocumentElement.SelectSingleNode("/DistanceMatrixResponse/row/element/duration/text").InnerText;
Label3.ForeColor = System.Drawing.Color.Green;
Label3.Text = output;
}
}
}
catch (Exception ex)
{
Label2.ForeColor = System.Drawing.Color.Red;
Label2.Text = "Error during processing";
return;
}
}
Screen Shots
1. Normal View
2. Enter Source Location:
3. Enter Destination Location
4. Results : Distance and Travelling Time
5. Reset
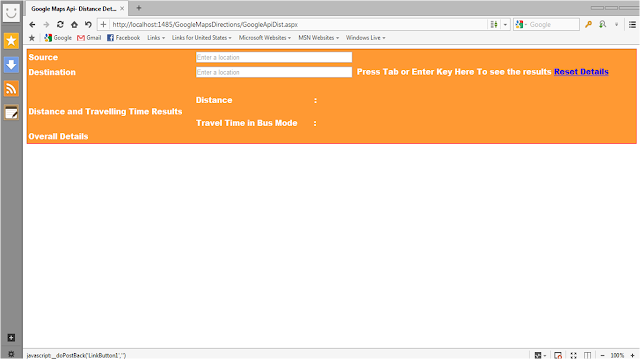
Code Behind Coding
Happy Coding,
Regards,
Kumaran K.
Comments
Post a Comment
Share this to your friends